What Is CherryPy?
CherryPy is an object-oriented Python framework developed to create web applications. It came out in 2002 and takes a different, interesting approach to its job. In this post, I’ll familiarize you with the basics of CherryPy, how it functions, and its advantages. By the end of this tutorial, you’ll understand what CherryPy is, how you can use it, and why it’s a great choice for building custom web applications.
Bird’s-Eye View of CherryPy
CherryPy is designed on the concept of multithreading. This gives it the benefit of handling multiple tasks at the same time. It also takes a modular approach, following the Model View Controller (MVC) pattern to build web services; therefore, it’s fast and developer-friendly.
Moreover, the framework uses core Python libraries, which mitigates the learning curve for developers who are well versed with Python. It supports Python’s latest versions from 3.5 to 3.8.
The concept of an object-oriented engine forms the core part of CherryPy’s working mechanism.
4 Advantages of CherryPy
At present there are a ton of Python frameworks you can use, especially for creating web applications. So what’s special about CherryPy? Here are a few points that make it distinguishable from its counterparts.
- Quick learning curve. The framework uses Python’s built-in conventions, allowing you to get started with basic Python knowledge. Just like regular Python applications, it provides extensive tools and plugins for faster development. Almost every framework has its own set of tools for computations, but CherryPy can also use existing Python libraries if necessary. If you or your team is well versed with Python, using CherryPy for your web service would give you a great time to market.
- Flexibility. The major advantage of using CherryPy over other Python-based frameworks like Django is that it doesn’t force the developers to stick to a particular structure, which makes it more flexible. You have more control and freedom over how to design your services, best practices to follow, etc.
- Versatility. CherryPy has the ability to create web services like RESTful Web Service (RWS), WSDL, SOAP, etc. You can use it to build things like e-commerce websites or authentication services by integrating various other Python modules.
- Affordable. CherryPy can easily be deployed in a cost-effective manner, as it has its own HTTP server for hosting an application on multiple types of gateways.
How to Install CherryPy
To install CherryPy in your system, you must have Python 3 and pip installed. If you’re using easy_install package manager, you can install CherryPy by running:

And here’s how you can do the same via pip:

Once installed, you can check the version installed by importing the module in the Python shell:

If the framework is installed correctly, it will show the version on the terminal.
Architecture and Working With CherryPy
The architecture of CherryPy consists of three integral components:
- Cherrypy.engine. This manages your website’s behavior, how the HTTP server functions (start/stop), and handles process reloads, file management, etc. However, the two important functions are:
- Creation of request and response objects
- Control and management of the entire process
- Cherrypy.server. This component configures and controls your actual HTTP server.
- Cherrypy.tools. This consists of various tools to help process HTTP requests. Remember I said CherryPy provides some great tools and plugins out of the box that speed up the development process? We can use these tools with the tools component in CherryPy.
Now that we’ve installed CherryPy on our local machines and have a fair idea of its architecture, let’s write our first line of code. The first step to start with the framework is to create a simple HTTP server. So, inside a directory of your choice, create a new .py file and add the following code:

Great! Let’s extend this further in the next section, where we’ll discuss how to create a web service using CherryPy.
Create a Web Service With CherryPy
Let’s go ahead and build a simple web application to manage a movie collection.
We will start by creating a movie_app.py file.
Now it’s time to start coding. The first step is to import CherryPy:

Next, we’ll define a movie database, which will consist of movies and their respective details. The data structure used will be a simple Python dictionary:
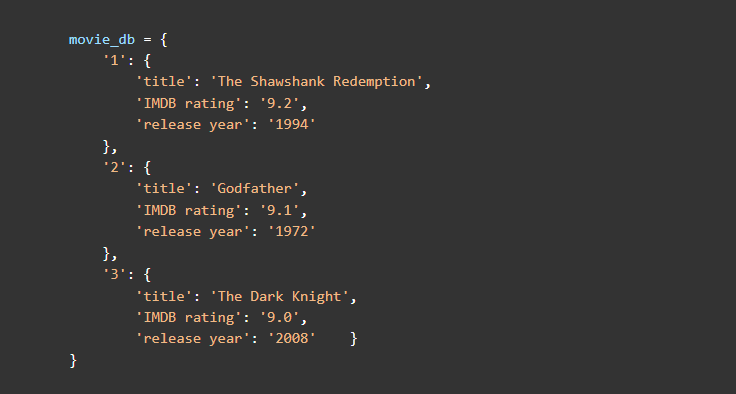
The next step is to create a class to access the movie database:
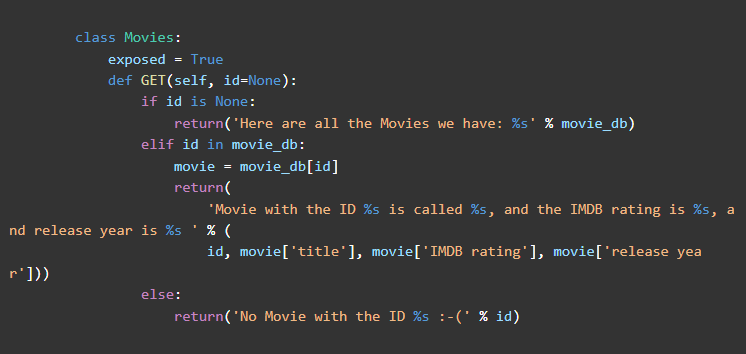
Now it’s time to create the main function.
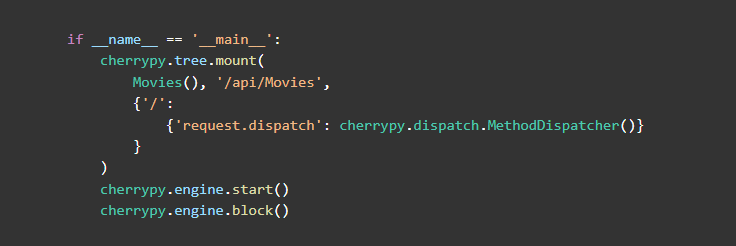
This function consists of an object for the class and links it to the URL ‘/api/Movies’. The app will handle all the requests coming to the URLs, which will begin with /api/Movies.
The MethodDispatcher is a tool in CherryPy that connects HTTP requests to handlers automatically.
The engine start and block command is written for the server.
Let’s run the application. You can use the command python movie_app.py. If all goes well, you should get the messages below on the terminal.

Now let’s test the application. Click on the link generated (in this case, http://127.0.0.1:8000) and type the URL /api/Movies.

When you visit the above URL, you should get the following JSON data back:

You can also see a particular movie’s data by adding the respective ID in the URLl as shown:

It should return you a single movie pertaining to that ID.

The presentation layer manages all the above interactions with each component. Now let’s learn more about what this layer is and how it functions.
Presentation Layer of CherryPy
This layer is responsible for proper communication between the sender and the targeted recipients. CherryPy manages the working of this layer through various templates. The role of the template engine is to take the input page and process the business logic required to produce the final output, which is the final page.
For instance, Kid is a simple template engine consisting of the name of the template and input of the processed data.
Tooling in CherryPy
As we know, CherryPy has its own set of tools. One of the tools is the Basic Authentication Tool, which provides authentication to the particular application under development.
After generating a username and password for the application, use this tool to implement them in the application. You can do this by activating the tool using the syntax shown below:

Conclusion
This should be enough to get you started building applications with CherryPy. Personally, I love how easy it is to create web services in CherryPy due to its architecture and working mechanism. Moving forward, I’d definitely recommend you create a full-blown web service like an authentication service with CherryPy. Until next time!
This post was written by Siddhant Varma. Siddhant is a full stack JavaScript developer with expertise in front-end engineering. He’s worked with scaling multiple startups in India and has experience building products in the Ed-Tech and healthcare industries. Siddhant has a passion for teaching and a knack for writing. He’s also taught programming to many graduates, helping them become better future developers.